# 介绍
弹出模态框,常用于消息提示、消息确认,或在当前页面内完成特定的交互操作,支持函数调用和组件调用两种方式。
# 引入
在app.json或index.json中引入组件,详细介绍见快速上手
"usingComponents": {
"mx-dialog": "/miniprogram_npm/m-ui/mx-dialog/index",
}
# 小程序码
可使用微信扫码查看小程序码
#
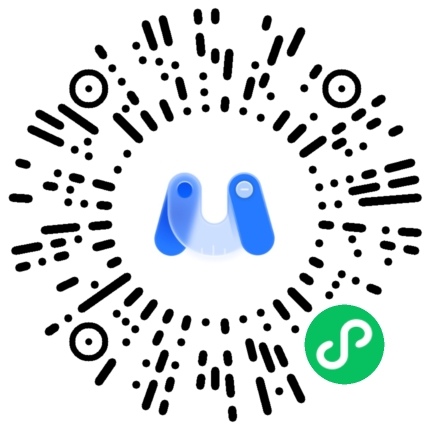
# 代码演示
# 基本用法
用于提示一些消息,只包含一个确认按钮。
<mx-dialog id="mx-dialog" />
import Dialog from '/miniprogram_npm/m-ui/dialog/dialog';
Dialog.alert({
title: '标题',
message: '弹窗内容',
}).then(() => {
// on close
});
Dialog.alert({
message: '弹窗内容',
}).then(() => {
// on close
});
# 消息确认
用于确认消息,包含取消和确认按钮。
<mx-dialog id="mx-dialog" />
import Dialog from '/miniprogram_npm/m-ui/dialog/dialog';
Dialog.confirm({
title: '标题',
message: '弹窗内容',
})
.then((res) => {
if (res.action == 'confirm') {
wx.showToast({
icon: 'none',
title: '点击确定',
})
}
// on confirm
})
.catch(() => {
if (error.action == 'cancel') {
wx.showToast({
icon: 'none',
title: '点击取消',
})
}
// on cancel
});
# 消息确认
用于确认消息,包含取消、确认、更多按钮。
<mx-dialog id="mx-dialog" />
import Dialog from '/miniprogram_npm/m-ui/dialog/dialog';
Dialog.confirmMore({
context: this,
zIndex: 10001,
title: '标题',
message,
})
.then((res) => {
console.log(res)
if (res.action == 'confirm') {
wx.showToast({
icon: 'none',
title: '点击确定',
})
}
// on confirm
if (res.action == 'more') {
wx.showToast({
icon: 'none',
title: '点击更多',
})
}
// on confirm
})
.catch((error) => {
if (error.action == 'cancel') {
wx.showToast({
icon: 'none',
title: '点击取消',
})
}
// on cancel
})
},
# 异步关闭
通过 beforeClose 属性可以传入一个回调函数,在弹窗关闭前进行特定操作。
<mx-dialog id="mx-dialog" />
import Dialog from '/miniprogram_npm/m-ui/dialog/dialog';
const beforeClose = (action) => new Promise((resolve) => {
setTimeout(() => {
if (action === 'confirm') {
resolve(true);
} else {
// 拦截取消操作
resolve(false);
}
}, 1000);
});
Dialog.confirm({
title: '标题',
message: '弹窗内容'
beforeClose
});
# API
# 方法
方法名 | 参数 | 返回值 | 介绍 |
---|---|---|---|
Dialog | options | Promise | 展示弹窗 |
Dialog.alert | options | Promise | 展示消息提示弹窗 |
Dialog.confirm | options | Promise | 展示消息确认弹窗 |
Dialog.confirmMore | options | Promise | 展示消息确认弹窗 |
Dialog.setDefaultOptions | options | void | 修改默认配置,对所有 Dialog 生效 |
Dialog.resetDefaultOptions | - | void | 重置默认配置,对所有 Dialog 生效 |
Dialog.close | - | void | 关闭弹窗 |
Dialog.stopLoading | - | void | 停止按钮的加载状态 |
# Options
通过函数调用 Dialog 时,支持传入以下选项:
参数 | 说明 | 类型 | 默认值 |
---|---|---|---|
title | 标题 | string | - |
width | 弹窗宽度,默认单位为px | string、 number | 270px |
message | 文本内容,支持通过\n换行 | string | - |
messageAlign | 内容对齐方式,可选值为 left right | string | center |
autoClose | 是否需要自动关闭 | boolean | true |
autoLoading | 不自动关闭时是否需要loading | boolean | false |
zIndex | z-index 层级 | number | 100 |
className | 自定义类名,dialog 在自定义组件内时无效 | string | '' |
customStyle | 自定义样式 | string | '' |
selector | 自定义选择器 | string | mx-dialog |
showConfirmButton | 是否展示确认按钮 | boolean | true |
showCancelButton | 是否展示取消按钮 | boolean | false |
showMoreButton | 是否展示更多按钮 | boolean | false |
confirmButtonText | 确认按钮的文案 | string | 确定 |
cancelButtonText | 取消按钮文案 | string | 取消 |
moreButtonText | 更多按钮文案 | string | 更多 |
overlay | 是否展示遮罩层 | boolean | true |
overlayStyle | 自定义遮罩层样式 | object | - |
closeOnClickOverlay | 点击遮罩层时是否关闭弹窗 | boolean | false |
beforeClose | 关闭前的回调函数,返回 false 可阻止关闭,支持返回 Promise | (action) => boolean | - |
context | 选择器的选择范围,可以传入自定义组件的 this 作为上下文 | object | 当前页面 |
transition | 动画名称,可选值为fade none | string | scale |
# Props
参数 | 说明 | 类型 | 默认值 |
---|---|---|---|
show | 是否显示弹窗 | boolean | - |
title | 标题 | string | - |
width | 弹窗宽度,默认单位为px | string 、 number | 270px |
message | 文本内容,支持通过\n换行 | string | - |
message-align | 内容对齐方式,可选值为 left right | string | center |
z-index | z-index 层级 | number | 100 |
class-name | 自定义类名,dialog 在自定义组件内时无效 | string | '' |
auto-close | 是否需要自动关闭 | boolean | true |
auto-loading | 不自动关闭时是否需要loading | boolean | false |
custom-style | 自定义样式 | string | '' |
show-confirm-button | 是否展示确认按钮 | boolean | true |
show-cancel-button | 是否展示取消按钮 | boolean | false |
show-more-button | 是否展示更多按钮 | boolean | false |
confirm-button-text | 确认按钮的文案 | string | 确定 |
cancel-button-text | 取消按钮文案 | string | 取消 |
more-button-text | 更多按钮文案 | string | 更多 |
confirm-button-color | 确认按钮的字体颜色 | string | #267AFF |
cancel-button-color | 取消按钮的字体颜色 | string | #267AFF |
more-button-color | 更多按钮的字体颜色 | string | #267AFF |
overlay | 是否展示遮罩层 | boolean | true |
overlay-style | 自定义遮罩层样式 | object | - |
close-on-click-overlay | 点击遮罩层时是否关闭弹窗 | boolean | false |
beforeClose | 关闭前的回调函数,返回 false 可阻止关闭,支持返回 Promise | (action) => boolean | - |
use-slot | 是否使用自定义内容的插槽 | boolean | false |
use-title-slot | 是否使用自定义标题的插槽 | boolean | false |
transition | 动画名称,可选值为fade none | string | scale |
scale | 动画scale时进入的缩放大小 | string | 0.7 |
# Event
事件名 | 说明 | 回调参数 |
---|---|---|
bind:close | 弹窗关闭时触发 | event.detail: 触发关闭事件的来源,枚举为confirm ,cancel ,more ,overlay |
bind:confirm | 点击确认按钮时触发 | - |
bind:cancel | 点击取消按钮时触发 | - |
bind:more | 点击更多按钮时触发 | - |
bind:overlay | 点击蒙层时触发 | - |
# Slot
名称 | 说明 |
---|---|
title | 自定义title显示内容,如果设置了title属性则不生效 |
← 蒙层(overlay) 弹窗(popup) →